This post is about my experience learning Rust by solving every CtCI problem live on Twitch, an unfinished project.


Rust is a modern systems-level programming language designed with safety in mind. It provides zero-cost abstractions, generics, functional features, and plenty more. I recently embarked on an effort to learn Rust properly, and I wanted to share some of my thoughts.
Until recently, I’d written only a handful of small programs in Rust, and after reading half of “Programming Rust”, I really didn’t know Rust. I figured a good way to get to know the language was to solve all 189 problems from the “Cracking the Coding Interview” book. Not only would I solve them with Rust, but I decided to do it live on Twitch. I’m no stranger to giving tech talks, or coding in front of an audience, but trying to learn a programming language, and explain what I was doing–live for the world to see–was something new for me.
Things started off a bit rough: technical hiccups, stream issues, tooling problems, and I had difficulty understanding the memory paradigm at first. Trying to do that, while also explaining what I was doing to people, was uh…tricky.
It took me about 8 hours to implement a linked list: I recorded two 4 hour streams of myself trying to figure out how to properly use Rc, RefCell, and Box. For a while I felt like I was just banging on the keyboard trying random combinations until something stuck. What’s amazing is that people tuned in to watch. I must have been doing something right.
After a bit of reading offline (and followed the very helpful “Learning Rust With Entirely Too Many Linked Lists” book), and the concepts started to click for me. After finishing my linked list implementation, things got easier.
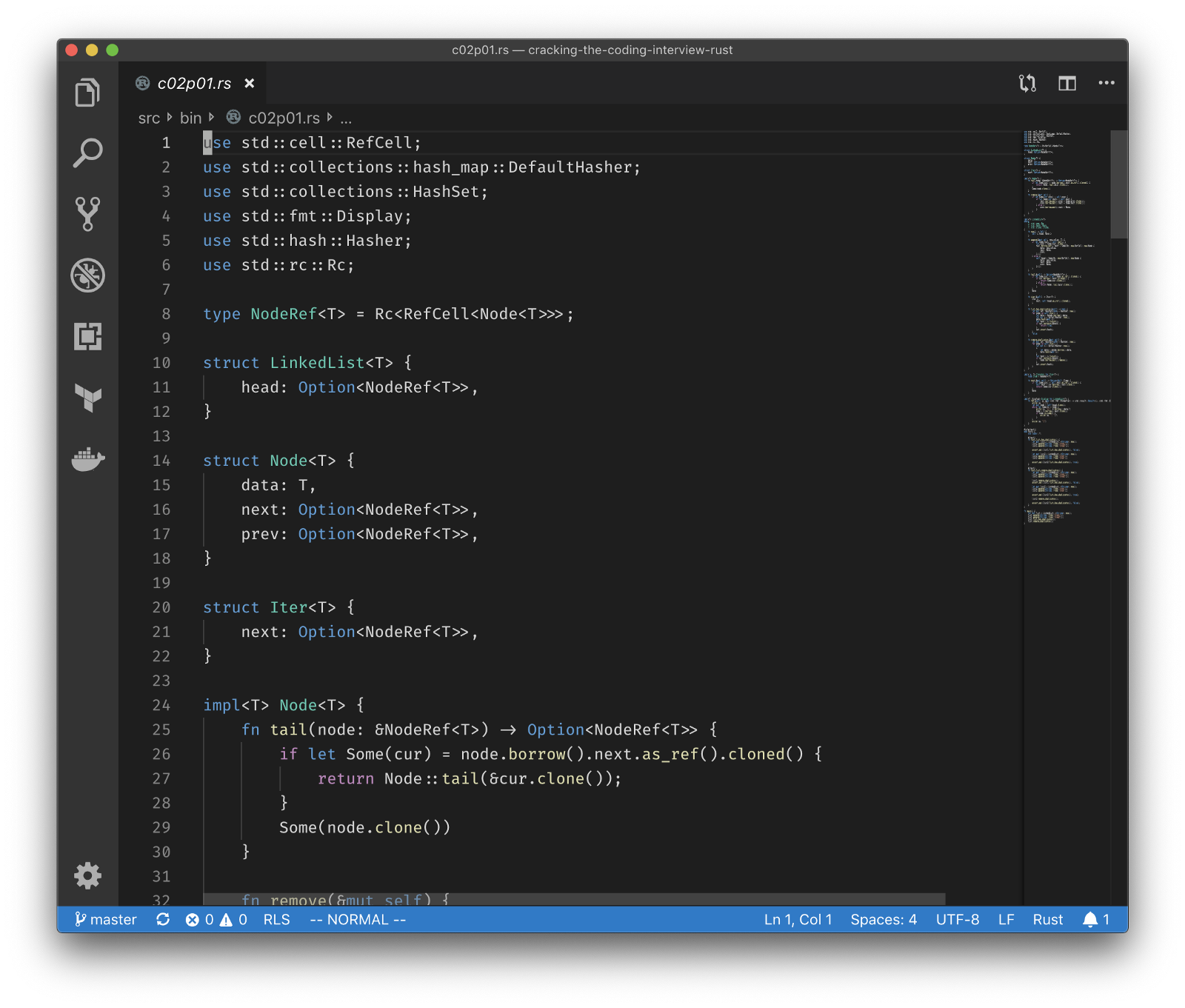
I’m now into chapter 4 of the book, and I feel like I’ve hit my stride. Rust feels natural, productive, and extremely satisfying once it compiles. Rust is strongly typed and provides excellent compiler messages: if you managed to appease the compiler, there’s a good chance your code will work–barring any logic flaws.
One lovely feature of Rust is how helpful the compiler can be. Compiler messages for C++ code, for example, are notoriously difficult to decipher. While Clang has made massive strides with its error messages, Rust’s compiler is another order of magnitude more helpful.
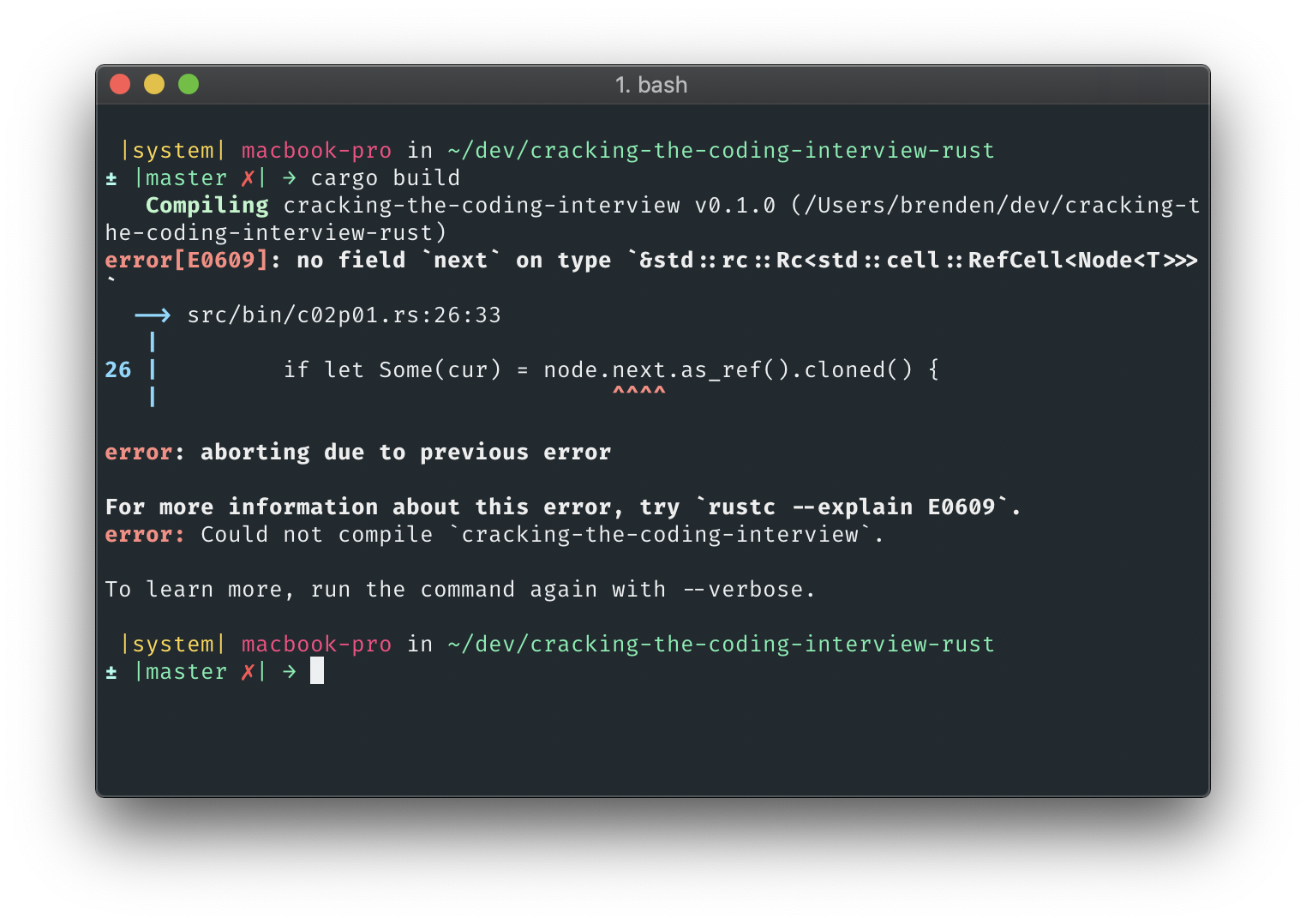
I’m going to summarize some of my findings thus far. This is based on my initial reactions, and I acknowledge my lack of Rust expertise, but it may still be interesting for others to see how their experience compares to mine. I woefully admit that I have not thoroughly researched every issue below, so I may have out of date or inaccurate information.
Language: The Good
First of all, kudos to the Rust team and everyone who has contributed to the project. This has been one of the most fun programming language learning experiences I’ve ever had. I don’t know if Rust will capture the mindshare of developers in the same way some other languages have, but I think it’s here to stay. On to the details:
- Rust code is fairly easy to read, and doesn’t suffer from the hard to parse syntax of languages like C++ or Scala. It seems to have what I expect it to have, and the challenge is just in figuring out which function to call.
- Having functional features like
map()
,filter()
,find()
, and so on are a delight. Defining higher order functions and passing closures to them is a breeze. It doesn’t make functional programming quite as easy as a language like Ruby, but it’s close. In fact, it’s amazing how easy it is for a language that performs comparably to C/C++. - Rust forces you to think hard about memory allocation, because you have no choice. In the end it means sloppy code is difficult to write, and good code is easy to write. These abstractions map directly to writing safe concurrent code as well.
- Rust’s zero-cost abstractions make it easy to write good code without adding overhead. Traits provide modern programming abstraction without the performance penalty.
- Rust code is safe (provided you don’t use the
unsafe
keyword, or call out to unsafe C libraries) - Rust’s
Result
andOption
provide a good way for dealing with functions that might return a value, or variables that might contain a value. A common pattern in C, C++, and even Java is for functions to return a null pointer when there’s nothing to return. In most cases, when this happens unexpectedly, it results in someone having a bad time.
Language: The Bad
- I find the need to
unwrap()
,as_ref()
andborrow()
a bit verbose at times. I wish there was some syntax sugar to cut down on the number of times I have to chain these calls together in different patterns. I find myself frequently writing code similar tooption.as_ref().unwrap().borrow()
, which feels icky. - There are certain trade offs the compiler needs to make in order to be able to compile code within a reasonable amount of time. As a result, there are some cases where
rustc
can’t infer a type, or it needs some human assistance in order to compile the code. For me, I’ve found it can sometimes be really difficult to figure out what the compiler needs, and why it can’t figure it out for me. - Some things occasionally feel too verbose. For example, converting between
str
andString
, or passing a reference instead of value to a function seems like something the compiler could figure out for me. I’m sure there’s a good reason for why it is the way it is, but it occasionally feels likerustc
is being too correct. - Having to handle every
Result
from every function is good; it means the programmer has to think about what’s happening with every function call. Sometimes it feels tedious. The?
operator can cut down on some of the verbosity, but there’s no good generalize way for handling the failure types. Crates like failure and error-chain make this easier, but you still need to explicitly define a case for every type of error that may occur.
Language: The Ugly
- Macros: WTF? Rust macros feel like a left turn compared to the rest of the language. To be fair, I haven’t been able to grok them yet, and yet they feel out of place like some strange bolt-on appendage, one which only came about after the language was designed, inspired by Perl. I will eventually take some time to understand them properly in the future, but right now I want to avoid them. Like the plague.
Tooling: The Good
- Rust provides decent tooling, and integrates with IDEs like VSCode through RLS. RLS provides support for linting, code completion, syntax checking, and formatting on the fly.
- Cargo is Rust’s powerful package manager: you’ll likely become familiar with it if you try Rust. For the most part, working with Cargo is a pleasure. There’s already a plethora of plugins for Cargo that provide additional features such as code coverage.
- Cargo is also a build system, and it can be used to run unit and integration tests. Configuring your build and dependencies is a snap with its somewhat declarative TOML syntax.
- Cargo integrates with crates.io which is the definitive source for open source Rust projects. Much like PyPi or RubyGems, you’ll find nearly all other Rust packages hosted on crates.io.
- rustup is the preferred tool for managing your Rust installation. You can select stable, beta, or nightly channels, and install specific builds from all previous releases. It also lets you install components like clippy and rustfmt
- clippy is a must have code linter if you’re a perfectionist like me. It will help you learn the Rust way, and it can catch many common mistakes that you might otherwise not notice. For me, clippy was helpful when I knew of a way to solve something, but I did not know the right way.
- rustfmt is an opinionated code formatter for Rust. In my opinion, opinionated formatters are the way to go. There’s no arguing about code formatting when everything adheres to the same standard.
- sccache, a compiler cache, will make things faster by reducing compile times. However — beware — sccache does not work with RLS, so you can’t use it with your IDE.
Tooling: The Bad
Okay, okay, before I go on about the problems with Rust, we should all acknowledge that this is a work in progress. The Rust tooling has come very far, very quickly, but I think it still has a long way to go. I’m going to highlight a few of the things that need improvement:
- Compilation feels slow. Not only is it slow, but I find I often have to recompile packages. I understand the necessity, but it’s still annoying at times. sccache helps, but it still feels slow. There are some ways to mitigate this, such as using
cargo check
instead ofcargo build
. - RLS uses racer for code completion, and I find that it’s hit or miss at best (in VSCode at least). Often functions that I expect to have completions for don’t exist, and functions that don’t exist show up as completion options. I haven’t done thorough analysis, but the suggestions seem to be right only about 75% of the time. The cause of this may simply be due to the slowness of RLS.
- No REPL: this may be unfair, since there’s no decent C++ REPL either, but a lot of languages come with a REPL these days. There’s an open issue on GitHub about this. A good REPL is not necessary, but would be helpful.
Tooling: The Ugly
- RLS is slow, buggy, and crashy. For me, at least, I find that I frequently need to restart RLS within VSCode. RLS is a great tool, but it does feel like it’s beta at best. I find myself having to pause and wait for RLS to catch up so I can make sure I’m not writing bad code. Sometimes I think it would be better to simply disable RLS, write the code, and then try and compile it like in the olden days when I did all my coding in Vim. It’s almost as if RLS has become too much of a crutch, and more of a distraction.
Libraries: The Good
- Surprisingly large number of available libraries in the Rust ecosystem. It seems as if there was a gold rush to run out and implement all the Rust libraries, and get your name immortalized in Rust history. You can find most of what you’d expect on crates.io or GitHub. I’m often surprised by how every search turns up 2 or 3 different implementations of what I’m looking for.
- Most libraries I have used work as expected, and many have exceeded expectations. This is a subtle and important distinction from the alternative, which are libraries which do not work.

Libraries: The Bad
- Although there are many libraries, I’ve found that a lot of them are incomplete, immature, or completely abandoned. The Rust community seems like it’s still in its early days, but it’s improving every day.
- Sometimes there are…too many options. For example, I wanted to use a logging library, and I discovered that there is a long list of options to choose from. Having many options is fine, but for something like this I just want to be told what to use. The Java ecosystem has a similar issue with java.util.logging, log4j, logback, log4j2, slf4j, and tinylog. To this day I still don’t know which Java logging library is the right one to use. With Rust, I just decided to use
env_logger
because it’s the first option in the list. - While not as bad as the Node.js ecosystem, the list of dependencies for every library has become quite long. I wrote a small GitHub bot called LabHub, and I’m still surprised by how many dependencies get pulled in (181 crates, if you’re wondering). To me, this suggests fragmentation and duplication, which could perhaps be improved by slowly graduating some widely needed features into the standard library (something that C++ has done very slowly).
Libraries: The Ugly
- I noticed that, along with a long list of dependencies for a relatively simple app, I was compiling different versions of the same libraries multiple times. I think Cargo is trying to be clever in order to maintain backward compatibility; it’s making guesses about which libraries to include based on semantic versioning. The thing that worries me about this is what happens when you have a library which depends on an ancient, broken version of some other library which also has a vulnerability. I have no doubt that the Rust authors have already considered this, but it still seems strange. To be fair, dealing with dependencies of dependencies is a very tricky problem. Thankfully there’s a tree tool for Cargo which can help sort these things out, and then you can force a dependency to upgrade its dependencies.
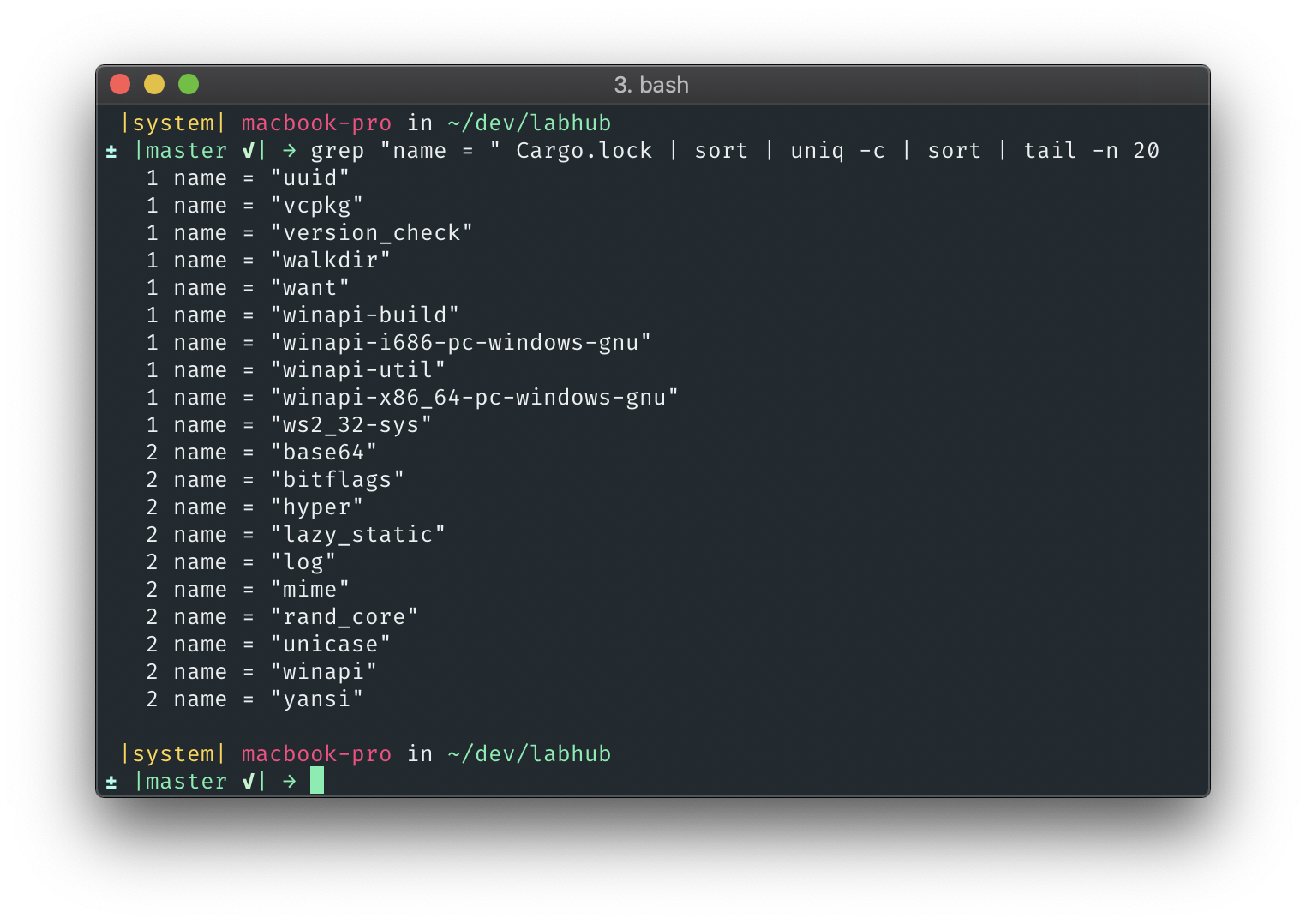
Final Thoughts
Rust is a great language. If programming is something you’re passionate about, please give it a shot. I hope you enjoy it.

About me: I’ve been writing code since I was 12 years old, have contributed to a number of different open source projects, and have worked at a variety of companies including Airbnb, Mesosphere, and Citadel. Find me on GitHub at github.com/brndnmtthws, Twitch at twitch.tv/brndnmtthws, or Twitter at twitter.com/brndnmtthws.